Static Maps API reference
Estimated reading time: 15 minutesThe Static Maps API lets you generate JawgMaps images from a base map and has the capability to draw geographic data on it.
Access token
Before accessing the Static Maps API, you need a valid access token. You can get your access tokens in your Jawg Lab account.
Requesting the Static Maps API
The Static Maps API supports multiple ways to request a static-map:
- Through a GET request where the parameters are provided in the query string
- Through a POST request where the same query string as above is sent in the request body as plain text
- Through a POST request with a JSON body
In this documentation examples will be provided with the GET and POST JSON versions.
Simple example
This simple example requests a static-map of Paris using either the GET API or the POST JSON API:
https://api.jawg.io/static?zoom=12¢er=48.856,2.351&size=400x300&layer=jawg-sunny&format=png&access-token={your-access-token}
// POST https://api.jawg.io/static?access-token={your-access-token}
{
"zoom": 12,
"center": {
"latitude": 48.856,
"longitude": 2.351
},
"size": {
"width": 300,
"height": 200
},
"layer": "jawg-sunny",
"format": "png"
}
All images in this documentation are actual calls to the Static Maps API.
Base Map Styles
The layer
parameter controls which base map style to use during the static-map rendering. Valid values are:
jawg-streets
default base map style with streets information.jawg-terrain
base map style with relief information.jawg-lagoon
blueish style inspired by lagoon.jawg-sunny
bright style.jawg-dark
dark style.jawg-light
light style.
Sizes
The size
parameter controls the dimensions of the requested static-map.
https://api.jawg.io/static?zoom=12¢er=48.856,2.351&size=600x400&layer=jawg-streets&format=png&access-token={your-access-token}
// POST https://api.jawg.io/static?access-token={your-access-token}
{
"zoom": 12,
"center": {
"latitude": 48.856,
"longitude": 2.351
},
"size": {
"width": 600,
"height": 400
},
"layer": "jawg-streets",
"format": "png"
}
Image Resolution
The scale
parameter controls the image resolution. Valid values are 1
(default) or 2
.
When you specify a scale
the static-map size
is multiplied by the scale
to determine the actual image size.
For example, if you specify a size
of 150x150
and a scale
of 2
, the image final size will be 300x300
.
https://api.jawg.io/static?zoom=15¢er=48.856,2.351&size=150x150&scale=2&layer=jawg-streets&format=png&access-token={your-access-token}
// POST https://api.jawg.io/static?access-token={your-access-token}
{
"zoom": 15,
"center": {
"latitude": 48.856,
"longitude": 2.351
},
"size": {
"width": 150,
"height": 150
},
"scale": 2,
"layer": "jawg-streets",
"format": "png"
}
The same area is covered but everything is bigger when scale
is 2
.
Center
The center
parameter controls the coordinates of the static-map center. This parameter take a pair of (latitude,
longitude) coordinates.
https://api.jawg.io/static?zoom=12¢er=44.837833,-0.5792061&size=300x300&layer=jawg-terrain&format=png&access-token={your-access-token}
// POST https://api.jawg.io/static?access-token={your-access-token}
{
"zoom": 12,
"center": {
"latitude": 44.837833,
"longitude": -0.5792061
},
"size": {
"width": 300,
"height": 300
},
"layer": "jawg-terrain",
"format": "png"
}
Zoom level
The zoom
parameter controls the static-map zoom level. The zoom level is an integer from 0 to 22 which defines the
level of details of the static-map.
Zoom 0
is the entire earth whereas zoom 20
can be used to focus on a single building.
https://api.jawg.io/static?zoom=5¢er=46.623399,2.437727&size=300x300&layer=jawg-terrain&format=png&access-token={your-access-token}
// POST https://api.jawg.io/static?access-token={your-access-token}
{
"zoom": 5,
"center": {
"latitude": 46.623399,
"longitude": 2.437727
},
"size": {
"width": 300,
"height": 300
},
"layer": "jawg-terrain",
"format": "png"
}
Image format
The static-map images can be rendered either in PNG or in JPEG format. This is controlled by the format
parameter.
Valid values are png
of jpg
.
The jpg
format provides smaller image size by doing a lossy compression which may degrade the image quality whereas
the png
format provides lossless compression at the cost of greater image size.
https://api.jawg.io/static?zoom=12¢er=48.856,2.351&size=300x300&layer=jawg-terrain&format=png&access-token={your-access-token}
// POST https://api.jawg.io/static?access-token={your-access-token}
{
"zoom": 12,
"center": {
"latitude": 48.856,
"longitude": 2.351
},
"size": {
"width": 300,
"height": 300
},
"layer": "jawg-terrain",
"format": "png"
}
Bounding Box
In the previous examples we used center
and size
parameters to place the static-maps views.
When you know the bounds of the view, you can use the bbox
parameter.
The query string format is bbox=west,south,east,north
.
For example: bbox=2.334938,48.835571,2.406864,48.879393
The JSON format is
{
"bbox": {
"w": 2.334938,
"s": 48.835571,
"e": 2.406864,
"n": 48.879393
}
}
Note that you still have to provide a zoom
level and the size of the static-map will be derived from the covered area
at that zoom level.
https://api.jawg.io/static?zoom=12&bbox=2.334938,48.835571,2.406864,48.879393&layer=jawg-dark&format=png&access-token={your-access-token}
// POST https://api.jawg.io/static?access-token={your-access-token}
{
"zoom": 12,
"bbox": {
"w": 2.334938,
"s": 48.835571,
"e": 2.406864,
"n": 48.879393
},
"layer": "jawg-dark",
"format": "png"
}
The covered area is the same but the sizes are different since we are more zoomed on the right.
Markers
The marker
parameters allows to add map pins to a static-map.
The query string format is marker=color:1392FC,size:small,label:A|-45.222,2.4444
.
You can repeat the marker
query parameter to add multiple markers to the static-map.
The JSON format is
{
"markers": [
{
"color": "1392FC",
"size": "small",
"label": "A",
"coords": {
"latitude": -45.222,
"longitude": 2.4444
}
}
]
}
The marker parameters values are:
color
RGB hex string color of the marker. (example:1392FC
)size
: specifies the marker size. Available values aresmall
,medium
orlarge
.label
: (optional) a single character that will be added to the marker. (example:A
)
The following example draws 2 markers A and B with different colors.
https://api.jawg.io/static?marker=color:1392FC,size:small,label:A%7C48.873325,2.290199&marker=color:9F9EFD,size:medium,label:B%7C48.872711,2.298428&zoom=15¢er=48.873798,2.294941&size=600x400&layer=jawg-streets&format=png&access-token={your-access-token}
// POST https://api.jawg.io/static?access-token={your-access-token}
{
"zoom": 15,
"center": {
"latitude": 48.873798,
"longitude": 2.294941
},
"size": {
"width": 600,
"height": 400
},
"markers": [
{
"color": "1392FC",
"size": "small",
"label": "A",
"coords": {
"latitude": 48.873325,
"longitude": 2.290199
}
},
{
"color": "9F9EFD",
"size": "medium",
"label": "B",
"coords": {
"latitude": 48.872711,
"longitude": 2.298428
}
}
],
"layer": "jawg-streets",
"format": "png"
}
Custom Icons
You can provide your own icons to replace the default marker icon. The custom icon marker parameters are:
icon
(required) the custom icon url (should be URL-encoded).anchor
(optional) specifies the icon anchor. Valid values arebottom
(default),center
,top
,left
,right
,topleft
,topright
,bottomleft
andbottomright
The color
, size
and label
parameters have no use in this context.
Supported formats for custom icons are PNG and JPEG. The icon sizes are limited to 8192
bytes.
The query string format is:
marker=icon:https://www.jawg.io/docs/images/icons/eiffel-tower.png,anchor:center|48.858257,2.294457
You can repeat the marker
query parameter to add multiple markers to the static-map.
Also, you might want to use URL-shortening services to reduce the length of the request URI which can quickly become
very long.
The JSON format is
{
"markers": [
{
"icon": "https://www.jawg.io/docs/images/icons/eiffel-tower.png",
"anchor": "center",
"coords": {
"latitude": 48.858257,
"longitude": 2.294457
}
}
]
}
The following example draws 3 custom icons of the Eiffel Tower, Big Ben and the Pisa Tower.
https://api.jawg.io/static?marker=icon:https://www.jawg.io/docs/images/icons/eiffel-tower.png,anchor:center%7C48.858257,2.294457&marker=icon:https://www.jawg.io/docs/images/icons/big-ben.png,anchor:center%7C51.500741,-0.124589&marker=icon:https://www.jawg.io/docs/images/icons/pisa-tower.png,anchor:center%7C43.722946,10.396612&size=600x400&layer=jawg-terrain&format=png&access-token={your-access-token}
// POST https://api.jawg.io/static?access-token={your-access-token}
{
"size": {
"width": 600,
"height": 400
},
"markers": [
{
"icon": "https://www.jawg.io/docs/images/icons/eiffel-tower.png",
"anchor": "center",
"coords": {
"latitude": 48.858257,
"longitude": 2.294457
}
},
{
"icon": "https://www.jawg.io/docs/images/icons/big-ben.png",
"anchor": "center",
"coords": {
"latitude": 51.500741,
"longitude": -0.124589
}
},
{
"icon": "https://www.jawg.io/docs/images/icons/pisa-tower.png",
"anchor": "center",
"coords": {
"latitude": 43.722946,
"longitude": 10.396612
}
}
],
"layer": "jawg-terrain",
"format": "png"
}

Note that we did not specify the zoom
and center
parameters. When using custom markers the Static Maps API will
determine the most suitable zoom level and center the static-map to see all markers.
See Zoom and Center Inference for more information.
Custom Icons and Scale
When using the scale=2
parameter, the custom icons are not scaled automatically by the Static Maps API as it
would result in a pixelated image. Instead you should supply a scaled version of your custom icons.

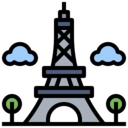
The following example draws a scaled custom icon of the Eiffel Tower and the images below show the difference between non-scaled and scaled version.
https://api.jawg.io/static?marker=icon:https://www.jawg.io/docs/images/icons/[email protected],anchor:bottom%7C48.858257,2.294457&zoom=13&size=200x150&scale=2&layer=jawg-terrain&format=png&access-token={your-access-token}
// POST https://api.jawg.io/static?access-token={your-access-token}
{
"zoom": 13,
"size": {
"width": 200,
"height": 150
},
"scale": 2,
"markers": [
{
"icon": "https://www.jawg.io/docs/images/icons/[email protected]",
"anchor": "bottom",
"coords": {
"latitude": 48.858257,
"longitude": 2.294457
}
}
],
"layer": "jawg-terrain",
"format": "png"
}


Paths
The path
parameter allows to draw a track and control its style.
The path
parameters are:
color
ARGB or RGB hex string color of the path. (example:771292FC
or1392FC
)weight
an integer controlling the width of the line. (example:1
)
The query string format is
path=color:1392FC,weight:3|48.8590,2.3398|48.8609,2.3408|48.8627,2.3352|48.8638,2.3358
You can repeat the path
query parameter to add multiple paths to the static-map.
The JSON format is
{
"paths": [
{
"color": "1392FC",
"weight": 3,
"coords": [
{
"latitude": 48.859,
"longitude": 2.3398
},
{
"latitude": 48.8609,
"longitude": 2.3408
},
{
"latitude": 48.8627,
"longitude": 2.3352
},
{
"latitude": 48.8638,
"longitude": 2.3358
}
]
}
]
}
The following example draws a 4 locations path near the Louvre Museum.
https://api.jawg.io/static?path=color:1392FC,weight:3%7C48.8590,2.3398%7C48.8609,2.3408%7C48.8627,2.3352%7C48.8638,2.3358&size=600x400&layer=jawg-streets&format=png&access-token={your-access-token}
// POST https://api.jawg.io/static?access-token={your-access-token}
{
"size": {
"width": 600,
"height": 400
},
"paths": [
{
"color": "1392FC",
"weight": 3,
"coords": [
{
"latitude": 48.8590,
"longitude": 2.3398
},
{
"latitude": 48.8609,
"longitude": 2.3408
},
{
"latitude": 48.8627,
"longitude": 2.3352
},
{
"latitude": 48.8638,
"longitude": 2.3358
}
]
}
],
"layer": "jawg-streets",
"format": "png"
}
Polylines
The path
locations can be encoded as
an encoded polyline instead of the "
pipe" |lat,lng|lat,lng|...
version. Polylines are much more compact making them relevant when the number of locations
in your path
becomes large.
The query string format is
path=color:1392FC,weight:3|yweiHe_hMgKaEkJ`b@}EaC
The polyline should be URL-encoded.
You can repeat the path
query parameter to add multiple paths to the static-map.
The JSON format is
{
"paths": [
{
"color": "1392FC",
"weight": 3,
"coords": "yweiHe_hMgKaEkJ`b@}EaC"
}
]
}
The following example draw a track of the Ascent of Mont Ventoux containing 124 locations encoded as a polyline.
https://api.jawg.io/static
?path=color:1392FC,weight:3%7C%7BqilG%7Dyq%5EbC%7BLnCqK%7CC%7DLvByOrBoMjBaL~FgHhDeMxC%7BN%7CDiMtD_LiD_GoBuLi%40aNd%40mM%5BaMsBuLaEaK%7BAkLpBmM%60DeLnAwLIgMb%40cM%7B%40wLbDiJrFeIvDaKVqNjEcJdIuCnFn%40yFqGgGoFvBuJcFeH_GwFyGeDgHyB%7DF%7BBiEcIgEuIyAyK_%40iL%60%40kK~%40cLRcLt%40gLiD_JgDcJwBkKsA%7DK%7CAqKbAkKwBeKc%40iLkAuKmDaFkCsCmB_K%7BByJ%7BEuG%7DCaJiCgIiDaJsDaJ_BiKm%40oKAt%40%7BCeK%3FiKEgLkDeIqEcHwBsHu%40kKy%40%7DE%7DDsIyEoG%7DEiH%7BG%7C%40sFvCqHISjKeHyAaHmCmHeD_HyE%40%60IlBxKw%40zHeHgDyGsDoAvG%7DBrHmHqDcDrDx%40jLcDvIwFzAcAvDx%40hLmB~KyDfJoFuB%7DFyBcDvJuCvJeD%7CIkGdENfKeAbKoHlBhAtHSfLuDtIqFbGmHvAuH%60AFjKbC%60Kn%40pKeAw%40
&size=600x400
&layer=jawg-terrain&format=png
&access-token={your-access-token}
// POST https://api.jawg.io/static?access-token={your-access-token}
{
"size": {
"width": 600,
"height": 400
},
"paths": [
{
"color": "1392FC",
"weight": 3,
"coords": "{qilG}yq^bC{LnCqK|C}LvByOrBoMjBaL~FgHhDeMxC{N|DiMtD*LiD_GoBuLi@aNd@mM[aMsBuLaEaK{AkLpBmM`DeLnAwLIgMb@cM{@wLbDiJrFeIvDaKVqNjEcJdIuCnFn@yFqGgGoFvBuJcFeH_GwFyGeDgHyB}F{BiEcIgEuIyAyK*@iL`@kK~@cLRcLt@gLiD_JgDcJwBkKsA}K|AqKbAkKwBeKc@iLkAuKmDaFkCsCmB_K{ByJ{EuG}CaJiCgIiDaJsDaJ_BiKm@oKAt@{CeK?iKEgLkDeIqEcHwBsHu@kKy@}E}DsIyEoG}EiH{G|@sFvCqHISjKeHyAaHmCmHeD_HyE@`IlBxKw@zHeHgDyGsDoAvG}BrHmHqDcDrDx@jLcDvIwFzAcAvDx@hLmB~KyDfJoFuB}FyBcDvJuCvJeD|IkGdENfKeAbKoHlBhAtHSfLuDtIqFbGmHvAuH`AFjKbC`Kn@pKeAw@"
}
],
"layer": "jawg-terrain",
"format": "png"
}
Note that we did not specify the zoom
and center
parameters. When using paths the Static Maps API will determine
the most suitable zoom level and center the static-map to see all paths.
See Zoom and Center Inference for more information.
Regions
The region
parameter allows to draw a polygon and control its style.
The region
parameters are:
color
ARGB or RGB hex string color of the polygon outline. (example:1392FC
)fillColor
ARGB or RGB hex string color to use to fill the polygon. (example:771392FC
)weight
an integer controlling the width of the outline. (example:3
)
The query string format is
region=color:1392FC,fillColor:771392FC,weight:3|48.8571,2.3523|48.8559,2.3516|48.8556,2.3527|48.8569,2.3533
You can repeat the region
query parameter to add multiple regions to the static-map.
The JSON format is
{
"regions": [
{
"color": "1392FC",
"fillColor": "771392FC",
"weight": 3,
"coords": [
{
"latitude": 48.8571,
"longitude": 2.3523
},
{
"latitude": 48.8559,
"longitude": 2.3516
},
{
"latitude": 48.8556,
"longitude": 2.3527
},
{
"latitude": 48.8569,
"longitude": 2.3533
}
]
}
]
}
Note that the first and last location not need to be the same to form a closed polygon. The Static Maps API will automatically close the polygon.
The following example draws a polygon over the Paris Hôtel de Ville. The polygon has a solid blue outline. The same color with some transparency is used to fill the polygon.
https://api.jawg.io/static?region=color:1392FC,fillColor:771392FC,weight:3%7C48.8571,2.3523%7C48.8559,2.3516%7C48.8556,2.3527%7C48.8569,2.3533&size=600x400&layer=jawg-streets&format=png&access-token={your-access-token}
// POST https://api.jawg.io/static?access-token={your-access-token}
{
"size": {
"width": 600,
"height": 400
},
"regions": [
{
"color": "1392FC",
"fillColor": "771392FC",
"weight": 3,
"coords": [
{
"latitude": 448.8571,
"longitude": 2.3523
},
{
"latitude": 48.8559,
"longitude": 2.3516
},
{
"latitude": 48.8556,
"longitude": 2.3527
},
{
"latitude": 48.8569,
"longitude": 2.3533
}
]
}
],
"layer": "jawg-streets",
"format": "png"
}
Polylines
The region
locations can be encoded as
an encoded polyline instead of the "
pipe" |lat,lng|lat,lng|...
version. Polylines are much more compact making them relevant when the number of locations
in your region
becomes large.
The query string format is
region=color:1392FC,fillColor:771392FC,weight:3|gleiHamjMpFdCr@uEsFcCq@rE
The polyline should be URL-encoded.
You can repeat the region
query parameter to add multiple regions to the static-map.
The JSON format is
{
"regions": [
{
"color": "1392FC",
"fillColor": "771392FC",
"weight": 3,
"coords": "yweiHe_hMgKaEkJ`b@}EaC"
}
]
}
The following example draws the boundaries of Paris that contains 29 locations encoded as a polyline.
https://api.jawg.io/static
?region=color:1392FC,fillColor:771392FC,weight:3%7C%7BzmiHibdMuH%7BvKhU_%7C%40xeAsWzwAcyAzrCeRxzApX%7BFy%7C%40yb%40md%40qSiyB%60PkaB~v%40%7BX%7CvAn%5DdDpvCuy%40riErHh%7DA%60%7C%40tgDkChgEi~%40bwFm%60%40dqAp%5Bbf%40aj%40hmBcbAh%5Byp%40%7CcDe%60B_n%40weAafDbN%7BuB%7BfAsvAucAkpD
&size=600x400
&layer=jawg-streets&format=png
&access-token={your-access-token}
// POST https://api.jawg.io/static?access-token={your-access-token}
{
"size": {
"width": 600,
"height": 400
},
"regions": [
{
"color": "1392FC",
"fillColor": "771392FC",
"weight": 3,
"coords": "{zmiHibdMuH{vKhU_|@xeAsWzwAcyAzrCeRxzApX{Fy|@yb@md@qSiyB`PkaB~v@{X|vAn]dDpvCuy@riErHh}A`|@tgDkChgEi~@bwFm`@dqAp[bf@aj@hmBcbAh[yp@|cDe`B_n@weAafDbN{uB{fAsvAucAkpD"
}
],
"layer": "jawg-streets",
"format": "png"
}
Note that we did not specify the zoom
and center
parameters. When using regions the Static Maps API will
determine the most suitable zoom level and center the static-map to see all regions.
See Zoom and Center Inference for more information.
Zoom and Center Inference
When providing marker
, path
or region
parameters, the Static Maps API can infer the center
and the zoom
level of the static-map so those 2 parameters become optional in this context. You can still provide them to force the
view of the static-map.
Note that the size
parameter must be provided for inference to work.
Automatic language
When you see a static maps from Jawg, it will be in your browser language. But you can force to a specific language for your website with a query parameter.
lang
(optional) specifies the lang for your static maps. Valid values arede
,en
,es
,fr
,it
,ja
,ko
,nl
,ru
,zh
. You can useint
for international map.
https://api.jawg.io/static
?size=400x300&zoom=4¢er=45,3
&format=png&lang=nl
&access-token={your-access-token}
// POST https://api.jawg.io/static?lang=nl&access-token={your-access-token}
{
"size": {
"width": 400,
"height": 300
},
"center": {
"latitude": 45,
"longitude": 3
},
"zoom": 4,
"format": "png"
}
Logo and attributions position
You can choose the position of the logo and OSM attributions on your static map with the query parameters logo
for
Jawg logo and attributions
for OSM attributions.
Attention, you must not obstruct our logo and OSM attributions, they must imperatively appear on the static maps! Logo
and OSM attributions can't have the same position.
logo
(optional) specifies the logo position. Valid values arebottomleft
(default),center
,top
,left
,right
,topleft
,topright
,bottom
andbottomright
attributions
(optional) specifies the OSM attributions position. Valid values arebottomright
(default),center
,top
,left
,right
,topleft
,topright
,bottomleft
andbottom
https://api.jawg.io/static
?size=400x300&zoom=4¢er=40,137
&layer=jawg-light&format=png
&logo=top&attributions=bottom
&access-token={your-access-token}
// POST https://api.jawg.io/static?access-token={your-access-token}
{
"size": {
"width": 400,
"height": 300
},
"center": {
"latitude": 40,
"longitude": 137
},
"zoom": 4,
"layer": "jawg-light",
"format": "png",
"logo": "top",
"attributions": "bottom"
}